Start developing
Fluent 2 provides a seamless maker experience from design to development to delivery.
Dive into the Fluent UI code libraries with these easy steps.
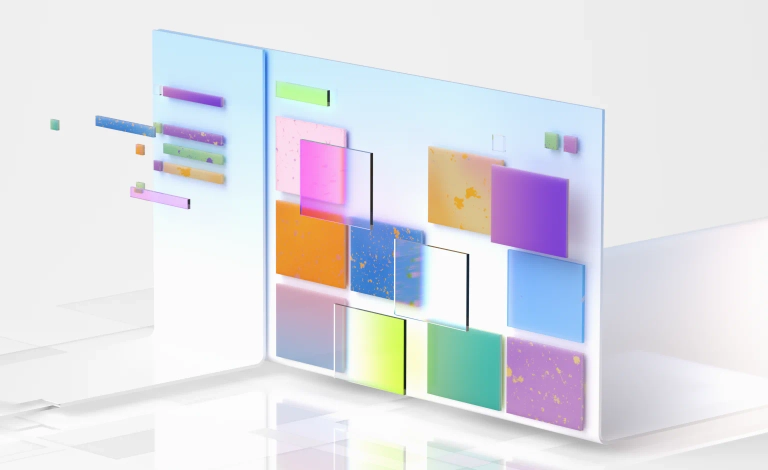
Pick your platform
Bring your app to your audience where and when they need it. Fluent’s cohesive design language is carried through each platform-specific Fluent UI library to ensure you can build the same great experiences across one platform or across them all.
Tooling and requirements
To build Fluent 2 experiences on React, you’ll need Fluent UI React v9. Fluent UI React is built on React and Typescript. The components are styled using CSS in JS. We use Griffel to render styles and insert CSS into the DOM when needed.
You’ll need node.js and a package manager like yarn to build and run apps using v9.
Migrating to Fluent UI React v9
Migrating from an older version of Fluent UI React? Check out our Migration topic in Storybook.
Installing Fluent UI React v9
Use your preferred package manager to install Fluent UI React as a dependency in your project.
npm install @fluentui/react-components
yarn add @fluentui/react-components
Setting up your app
- Import a FluentProvider and theme at the root.
-
Pass the theme as a prop of the
FluentProvider
. This defines your app-level settings. - Import any other v9 components you’ll need to render within the
FluentProvider
.
import {
FluentProvider,
webLightTheme,
Button
} from "@fluentui/react-components";
export default function App() {
return (
<FluentProvider theme={webLightTheme}>
<Button appearance="primary">Hello Fluent UI React</Button>
</FluentProvider>
);
}
Ready, set, make
You’re all set! Be sure to read our component documentation to create fully accessible and delightful experiences with Fluent.
Questions or feedback? Reach out to the team on GitHub.
Tooling and requirements
To build Fluent 2 experiences on iOS, you’ll need Fluent UI Apple. Fluent UI Apple contains native UIKit and AppKit controls for implementing the Fluent design system for iOS, iPadOS, and MacOS. Fluent UI Apple is built on Swift and supports Objective-C. To get started, you’ll need:
- iOS 14 or later or MacOS 10.15 or later
- Xcode 14.1 or later
- Swift 5.7.1 or later
Migrating to Fluent 2
With each release of Fluent UI Apple, we align more components to the Fluent 2 design language. Once a component is tokenized to align to Fluent 2, the Fluent 1 version will no longer be available.
Be sure to check the release notes to see what’s been changed or added. If you need to stick with the Fluent 1 version of an upgraded component, take the release before that component was tokenized.
Installing Fluent UI Apple
Using Swift package manager
To install FluentUI using SwiftUI, specify fluentui-apple as a dependency in your Xcode project or Package.swift
file.
dependencies: [
.package(url: "https://github.com/microsoft/fluentui-apple.git",
.upToNextMinor(from: "X.X.X")),
]
Using CocoaPods
To install Fluent UI Apple using CocoaPods, specify Fluent UI in your Podfile. For more info, see their getting started guide.
pod 'MicrosoftFluentUI', '~> X.X.X'
Installing manually
To manually install Fluent UI Apple into an existing Xcode project, download the latest changes from the FluentUI
Apple repository and move the fluentui-apple
folder into your project folder.
Moving Fluent UI Apple into your project folder
- Move ios/FluentUI.xcodeproj into your Xcode project
- In Xcode, select your project > your target > General > Frameworks, Libraries, and Embedded Content
- Add
libFluentUI.a
Setting up a demo environment
- To set up demo environments, select your project > your target > Build Phases > Copy Bundle Resources
- Add
FluentUIResources-iOS.bundle
Importing Fluent UI Apple
After installing the Fluent UI Apple library, import it to access Fluent components.
For Swift
import FluentUI
For Objective-C
#import <FluentUI/FluentUI-Swift.h>
Ready, set, make
You’re all set! Be sure to read our component documentation to create fully accessible and delightful experiences with Fluent.
Questions or feedback? Reach out to the team on GitHub.
Tooling and requirements
To build Fluent 2 experiences on Android, you’ll need Fluent UI Android. Fluent UI Android contains native UIKit and AppKit controls for implementing the Fluent design system for Android. Fluent UI Android is built on Kotlin. To get started, you’ll need:
- Android API 21 or later
- Android CompileSDK 33
- Kotlin 1.8.21
- Jetpack Compose BOM 2023.06.01
- Jetpack Compose Compiler 1.4.7
- Duo version 1.0.0-alpha01
- Android Studio Flamingo
Migrating to Fluent 2
With each release of Fluent UI Android, we align more components to the Fluent 2 design language. Once a component is tokenized to align to Fluent 2, the Fluent 1 version will remain in the same module, but the module will be separated into a tokenized and non-tokenized folder.
Be sure to check the release notes to see what’s been changed or added.
Installing Fluent UI Android
Using Gradle
Our library is published using MavenCentral, so make sure the mavenCentral()
repository has been added to your project level build.gradle file if you want to consume version 0.0.17 or later.
If you’re using older versions you can consume jcenter()
, too. Note, it will only work for
version 0.0.16 or earlier.
Inside the dependency block in your build.gradle, add a line for the FluentUI library, making sure to replace
$version
with the latest version of FluentUI.
dependencies {
...
implementation 'com.microsoft.fluentui:FluentUIAndroid:$version'
...
}
Starting at version 0.0.12, applications cal use individual modules as needed. For example, the BottomSheet component
is in the fluentui_drawer module. To use the BottomSheet without taking a dependency on other modules, add the
fluentui_drawer module to build.gradle. Replace $version
with the latest version of fluentui_drawer.
Learn more about the contents of each module and modularization in GitHub.
dependencies {
...
implementation 'com.microsoft.fluentui:fluentui_drawer:$version'
...
}
Develop for Surface Duo
Enhance your apps for dual-screen experiences with the Surface Duo SDK. To get the SDK, add the following lines to the repositories section in your gradle script.
maven {
url "https://pkgs.dev.azure.com/MicrosoftDeviceSDK/DuoSDK-Public/_packaging/Duo-SDK-Feed/maven/v1"
}
Add the SDK dependency to the module-level build.gradle file. Be sure to check the current version of the SDK; it may be different from what's shown here.
implementation "com.microsoft.device:dualscreen-layout:1.0.0-alpha01"
Using Maven
To install Fluent UI Android using Maven, add the FluentUI library as a dependency. Make sure to replace ${version}
with the latest version of FluentUI:
<dependency>
<groupId>com.microsoft.fluentui</groupId>
<artifactId>FluentUIAndroid</artifactId>
<version>${version}</version>
</dependency>
Applications can use specific modules as needed. Make sure to replace ${version}
with the
latest version of the component you need.
Learn more about the contents of each module and modularization in GitHub.
<dependency>
<groupId>com.microsoft.fluentui</groupId>
<artifactId>fluentui_drawer</artifactId>
<version>${version}</version>
</dependency>
Installing manually
To manually install Fluent UI Android into an existing Xcode project, download the latest changes from the Fluent UI Android repository. Modularized FluentUI manual installation involves building individual AAR files of required modules.
Building AAR files from FluentUI Modules
Follow these instructions to build and output an AAR file from the FluentUI modules, import the module(s) to your project, and add it as a dependency. If you're having trouble generating an AAR file for the module, make sure you select it and run e.g "Make Module 'fluentui_drawer'" from the Build menu.
Some components have dependencies you will need to manually add to your app if you are using this library as an AAR artifact because these dependencies aren’t included in the output. Double check that these library versions correspond to the latest versions we implement in the FluentUI build.gradle.
If using PeoplePickerView, include this dependency in your gradle file:
implementation 'com.splitwise:tokenautocomplete:2.0.8'
If using CalendarView or DateTimePickerDialog, include this dependency in your gradle file:
implementation 'com.jakewharton.threetenabp:threetenabp:1.1.0'
Using the demo app
- A full list of implemented controls available in the demo can be found here: Demos.
- To see samples of all of our implemented controls and design language, run the FluentUI.Demo module in Android Studio.
Importing Fluent UI Android
After installing the Fluent UI Android library, import it to access Fluent components.
Import and use the library
In code for importing the entire library:
import com.microsoft.fluentui
In code for a single component import:
import com.microsoft.fluentui.persona.AvatarView
In XML:
<com.microsoft.fluentui.persona.AvatarView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:name="Mona Kane" />
Ready, set, make
You’re all set! Be sure to read our component documentation to create fully accessible and delightful experiences with Fluent.
Questions or feedback? Reach out to the team on GitHub.
Fluent in WinUI
To get the building blocks for crafting Windows experiences, use WinUI. These components incorporate Fluent’s design language, so you can be confident you’re building great experiences within the Fluent ecosystem.